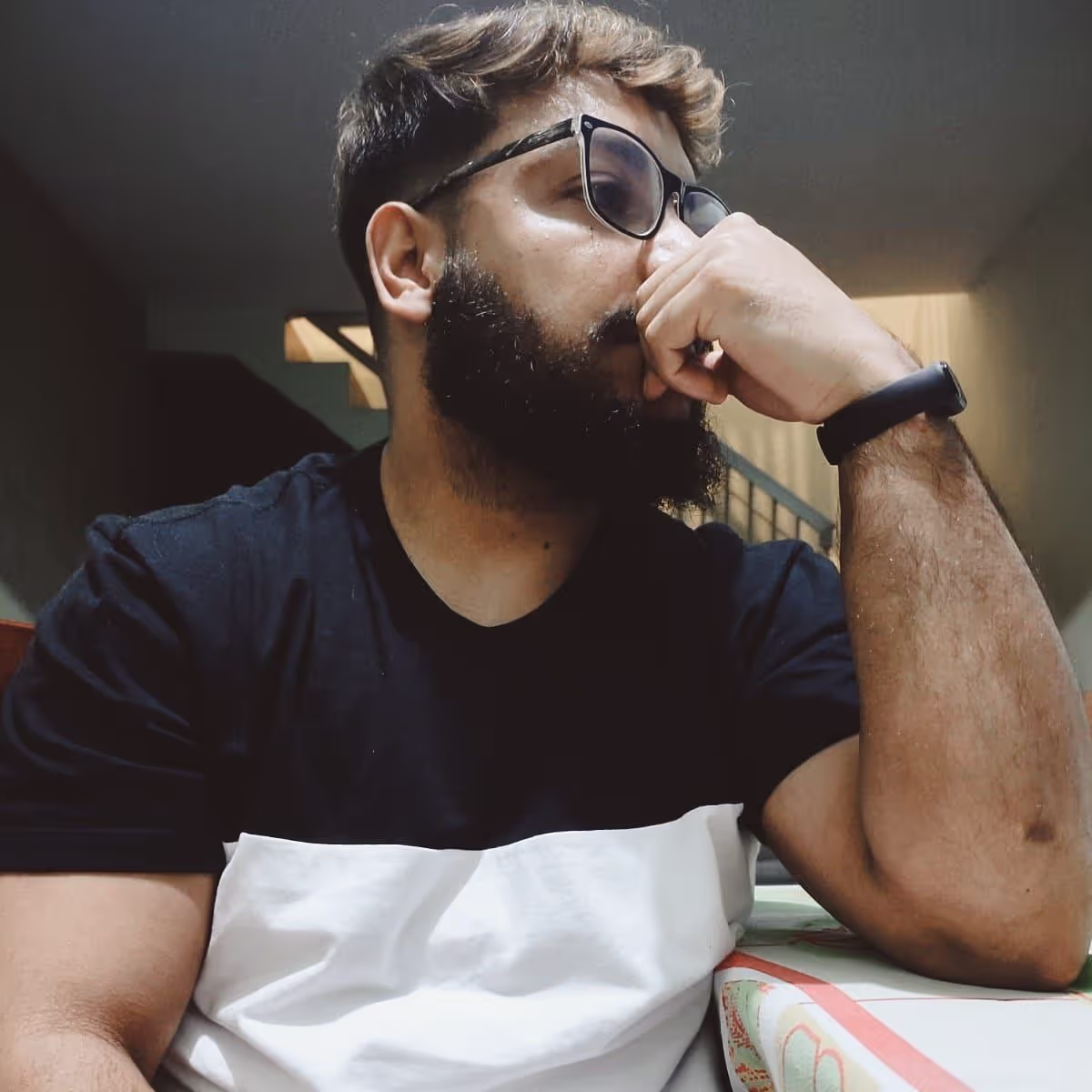
Simão Júnior
Software Developer in São Paulo, Brazil
Hi, I’m Simão Júnior, a self-taught software engineer based in São Paulo. My main focus is backend development, where I build scalable, efficient systems and solve complex technical challenges. I currently work at Nextcode, developing Onboard, a platform that simplifies company onboarding processes.
Over the past 4 years, I’ve been focusing on backend technologies, working extensively with JavaScript, TypeScript, and Node.js. I’ve also been contributing to projects using Elixir and related tools. My passion lies in architecting robust backend solutions and infrastructure, with a particular interest in distributed systems.
Outside of work, I’m continuously learning across tech and other domains. I’m an avid reader of sci-fi and literature on digital products and technology. When I’m not coding, I enjoy spending time with my wife, Kelly and our cat, Yuki. I also enjoy going to the gym and occasionally wrestle with NixOS (I use NixOS BTW).
I’m always open to new collaborations and technical discussions. Let’s connect!
- Email [email protected]
- GitHub simaojunior
- Website Simão Júnior
- 2021 - NowSofware Engineer Nextcode
Currently building a onboard platform. Responsibilities include:
- Build new features into the app using NodeJS and Typescript.
- Maintain the app and fix bugs.
- Work closely with the product team to understand the needs of the business and the users.
Tools used: NodeJS, VueJs, TypeScript, MongoDB, Redis, Nats, Docker and Microservices.